1. sass 安装
检查是否安装成功
1 2
| $ sass --version 1.42.1 compiled with dart2js 2.14.2
|
2. 把 sass 编译成 css
进入 sass 项目所在目录
项目结构
1 2 3 4 5
| C:sass_study ├─css │ └─scss style.scss
|
编译 sass
1
| sass scss/style.scss:css/style.css
|
说明: 冒号左边为要编译的文件, 冒号右边为编译后的输出文件.
3. 自动编译 sass
可以使用–watch 选项监视一个目录, 当目录中的文件发生变化以后, 自动将变化的文件编译后保存到 css 目录
1 2
| $ sass --watch scss:css Sass is watching for changes. Press Ctrl-C to stop.
|
4. 修改编译输出的 css 格式
sass 输出有四种格式:
nested 嵌套
compact 紧凑
expanded 扩展
compressed 压缩
例如使用扩展, 这种样式常常用在开发模式下
1
| sass --watch scss:css --style expanded
|
5. .sass 和 .scss 的区别
从 sass3.0 以后 sass 的语法发生了较大的变化,
新的样式被称为 sassy css, 区别主要在以下方面.
首先这两种文件的扩展名不一样
两种文件的注释方式不一样
scss 语法比较接近 css, sass 是缩进式语法
scss import 是需要在文件名上添加引号, sass 不需要
scss mixin 需要使用@, sass mixin 需要使用前面需要=号
其他见下图
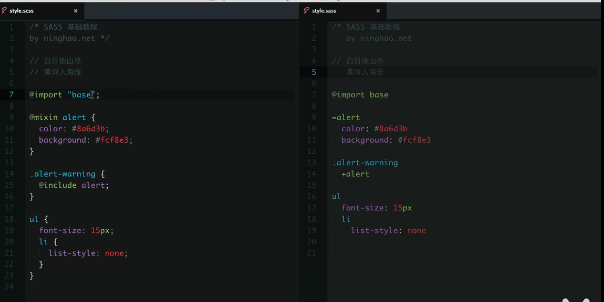
6. 变量 variables
声明变量以$开头, 冒号为赋值符号
1
| $primary-color: #123456;
|
使用变量
1 2 3
| div.box { backgound-color: $primary-color; }
|
1 2 3 4 5 6 7 8 9 10 11 12 13
| h1.pageheader { border: 1px solid $primary-color; } ``
变量的值可以是数字, 字符串, 颜色值, 如果是字符串的话需要使用引号引起了 例如 ```scss $primary-color: #123456; $primary-border: 1px solid $primary-color; h1.pageheader { border: $primary-border; }
|
变量名字中可以包含中划线(-), 也可以使用下划线(_), 最好是根据团队的编程规范进行统一命名规范.
7. 嵌套 Nesting
1 2 3 4 5 6 7 8 9 10 11
| .nav { height: 100px; ul { margin: 0; li { float: left; list-style: none; padding: 5px; } } }
|
以上嵌套写法对应的 css 输出样式如下
1 2 3 4 5 6 7 8 9 10 11
| .nav { height: 100px; } .nav ul { margin: 0; } .nav ul li { float: left; list-style: none; padding: 5px; }
|
伪类选择嵌套写法
1 2 3 4 5 6 7 8 9
| a { display: block; color: #820; padding: 5px; &:hover { background-color: #082f7e; color: #fff; } }
|
输出样式
1 2 3 4 5 6 7 8 9
| a { display: block; color: #820; padding: 5px; } a:hover { background-color: #082f7e; color: #fff; }
|
引用父选择器的例子
1 2 3 4 5 6
| .nav { height: 100px; & &-text { font-size: 15px; } }
|
css 输出
1 2 3 4 5 6
| .nav { height: 100px; } .nav .nav-text { font-size: 15px; }
|
8. 嵌套属性
示例 1:
1 2 3 4 5 6 7
| body { font: { family: Arial; size: 15px; weight: normal; } }
|
输出的 css
1 2 3 4 5
| body { font-family: Arial; font-size: 15px; font-weight: normal; }
|
示例 2:
1 2 3 4 5 6
| .nav { border: 1px solide #020 { left: 0; right: 0; } }
|
输出的 css
1 2 3 4 5
| .nav { border: 1px solide #020; border-left: 0; border-right: 0; }
|
9. 混合 mixin
可以把它想象成一块有名字的定义好的样式, 有点像 js 中的函数
定义 mixin 的语法
1 2 3
| @mixin 名字(参数1, 参数2 ...) { ... }
|
调用 mixin 的语法
@include 名字(参数 1, 参数 2 …)
说明如果 mixin 不带参数, 则调用方式如下
@include 名字
10. 继承/扩展 inheritance
关键字@extend
示例
1 2 3 4 5 6 7 8 9 10 11 12
| .alert { padding: 15px; }
.alert a { font-weight: bold; }
.alert-info { @extend .alert; background-color: #d9df7; }
|
输出
1 2 3 4 5 6 7 8 9 10 11 12 13
| .alert, .alert-info { padding: 15px; }
.alert a, .alert-info a { font-weight: bold; }
.alert-info { background-color: #d9df74; }
|
11. Partials 与 @import
partial 文件需要以下划线(_)开头
示例:
文件结构
├─css
│
└─scss
style.scss
_base.scss
_base.scss 内容
1 2 3 4
| body { padding: 0; margin: 0; }
|
style.scss 内容
注意: import 时不需要输入文件名前面的下划线, 也不需要输入文件的扩展名
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| @import "base";
.alert { padding: 15px; }
.alert a { font-weight: bold; }
.alert-info { @extend .alert; background-color: #d9df74; }
|
输出 css 如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| @import "base";
.alert { padding: 15px; }
.alert a { font-weight: bold; }
.alert-info { @extend .alert; background-color: #d9df74; }
|
12. scss 中的注释
scss 中有三种注释:
单行注释, 多行注释, 和强制注释
他们之间的差别为:
单行注释不会被编译到输出文件中,
多行注释一般情况下会输出到编译后的输出文件, 但是在压缩输出时会被去除掉, 强制注释会输出到编译后的 css 文件,在任何输出样式中都不会被清除.
单行注释示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| background-color: yellow; `` 多行注释 ```scss
background-color: yellow; ``
强制注释
```scss
background-color: yellow;
|
13. 数据类型 data type
sass -i 使用交互式模式
scss 属于类型有:
数值型: number
字符串: string
列表: list
颜色: color
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| $ sass -i >> type-of(5) >> number >> type-of(5px) >> number >> type-of(hello) >> string >> type-of(1px solid red) >> list >> type-of(10px 5px) >> list >> type-of(#123456) >> color >> type-of("hello") >> string >> type-of($colors) >> map
|
14. 数值运算
14.1. 加法运算
2+8 结果 10
5px + 5px 结果 10px
14.2. 减法
8-2 结果 6
5px -2 结构 3px
14.3. 乘法
28 结果 16
5px2 结果 10px
5px*2px 结果 10px*px
注意: 虽然有结果, 但是 px*px 不是 css 能识别的单位
14.4. 除法
(8/2) 结果 4
(10px/2) 结果 5px
注意: 除法需要加括号, 因为/已经是 css 值得关键字 例如 font: 16px/1.8 serif
混合运算
2+3*5px 结果 17px
15. sass 函数
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| $ sass -i >> abs(10) >> 10 >> abs(10px) >> 10px >> abs(10ox) >> 10ox >> type-of(10ox) >> number round(10.5) >> 11 cell(10.3) >> cell(10.3) min(1,2,4,3) >> 1
|
16. 字符串
字符串连接
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| $ sass -i >> hello + " world!" >> hello world! >> "hello" + world! >> ^ Error: Expected "important". >> "hello" + world >> "helloworld" >> "hello" world >> "hello" world >> "hello" / world >> "hello"/world >> hello* world ^^^^^^^^^^^^ Error: Undefined operation "hello * world". >> hello + world >> helloworld >> hello *2 ^^^^^^^^ Error: Undefined operation "hello * 2". >> hello - world >> hello-world
|
17. 字符串变量
1 2 3 4 5 6 7 8 9
| $ sass -i >> $greeting: "hello world" >> "hello world" >> $greeting >> "hello world" >> to_upper-case($greeting) >> "HELLO WORLD" >> str-length($greeting) >> 11
|
18. 颜色
1 2 3 4 5 6 7 8 9
| #123456
RGB(255, 0, 0) RGBA(255, 0, 0, 0.5) red
hsl(0, 100%, 50%) hsla(0, 100%, 50%, 0.2)
|
可以使用操作符处理颜色值, 也可以使用函数操作颜色
颜色操作函数:
lighten, darken: 增减颜色的亮度
1 2 3 4 5 6 7
| $base-color: hsl(222, 100%, 50%) $light-color: lighten($base-color, 30%) $dark-color: darken($base-color, 20%);
.alert { color: $dark-color; }
|
19. 列表
列表和列表函数
append
length
index
join
20. map 类型的数据
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| $ sass -i >> $colors: (light:#123456) >> (light: >> $colors: (light:#123456, dark:#000000) >> (light: $colors >> (light: >> length($colors) >> 2 >> map-get($colors, light) >> >> map-keys($colors) >> light, dark >> map-values($colors) >> map-has-key($colors, light) >> true map-merge($colors, (light-gray: >> (light:
|
21. boolean 值
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| $ sass -i >> 5px > 3px >> true >> 5px < 3px >> false >> (5px > 3px) and (4px < 3px) >> false >> (5px > 3px) and (4px > 3px) >> true >> (5px > 3px) or (4px < 3px) >> true >> (5px > 3px) or (4px > 3px) >> true >> (5px < 3px) or (4px < 3px) >> false not(5px> 3) >> false >> not(5px> 3px) >> false >> not(5px < 3px) >> true
|
22. 函数插值法(Interpolation)
把变量放到属性名称上面
1 2 3 4 5 6 7 8 9
| $version: "0.0.1"
$name: "info"; $attr: "border"
.alert-#($name) { #($attr)-color: #ccc }
|
css 输出:
1 2 3 4 5
| @charset "UTF-8";
.alert-info { border-color: #ccc; }
|
23. 控制指令
@if
@for
@each
24. if 指令
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| $use-prefixes: true; body { padding: 0; }
.rounded { @if $use-prefixes { -webkit-border-radius: 5px; -moz-border-radius: 5px; -ms-border-radius: 5px; -o-border-radius: 5px; }
border-radius: 5px; }
|
css 输出
1 2 3 4 5 6 7 8 9 10 11
| body { padding: 0; }
.rounded { -webkit-border-radius: 5px; -moz-border-radius: 5px; -ms-border-radius: 5px; -o-border-radius: 5px; border-radius: 5px; }
|
25. for 循环
1 2 3 4 5 6 7
| $columns: 12;
@for $i from 1 through $columns { .col-#{$i} { width: 100% / $columns * $i; } }
|
26. each 循环
1 2 3 4 5 6
| $icons: success error warning;
@each $icon in $icons { .icon-#{$icon} { } }
|
27. while 循环
28. 用户自定义函数
1 2 3
| 语法 @function 名称 (参数1, 参数2) { @return; }
|